When I was in grade-school math class, the teachers would always implore us to show our working. When answering a question, you wouldn’t always get to the right answer, but if you could show you’d approach the problem in the right way then there were still marks to be awarded.
You should take exactly the same approach answering programming problems in interviews.
Interviews are limited opportunities to learn about someone
As we covered in Can you pretend to be normal for up to two hours?, there’s not really that much that can be found out about someone in a technical interview.
The list basically comes down to:
- Can they answer questions about the skills they claim to have experience with?
- Can they present some evidence of their experience with those same skills?
- Are they capable of maintaining a façade of normality for at least a few hours?
- Can they – when motivated to – have a technical discussion where they respectfully listen to the other person’s opinion?
- Do you like them, basically?
Nowhere in the list is “have a dictionary knowledge of algorithms and perfectly solve all technical challenges”. Heck, three of our five bullet points deal entirely with just your inter-personal skills while answering technical questions. The other two deal with showing evidence that you know how to approach certain technologies.
The answer to any given technical question you ask is usually not that important. Getting to a right answer the wrong way — rote repetition of something you’ve memorized or just guessing around — is usually the wrong answer. Getting annoyed that you’ve being asked questions that you don’t think are appropriate is almost always the absolute worst answer.
Doing anything other than demonstrating how you think a programmer should approach unfamiliar technical challenges is the wrong answer. That’s what you’re really being tested on.
How to reverse a binary tree
Short interlude for one of my favourite tweets of all time:
Google: 90% of our engineers use the software you wrote (Homebrew), but you can’t invert a binary tree on a whiteboard so fuck off.
— Max Howell (@mxcl) June 10, 2015
Let’s say that unlike the author of that tweet, you actually want to get a job. And let’s say you’re asked in an interview, “How would you reverse a binary tree?”
I’ve got an MSc in Software Engineering from Oxford and reversing a binary tree is not something I’ve ever had to think about it before. So … let’s instead show off how a programmer approaches an unfamiliar technical problem (other than StackOverflow).
What don’t you know, and what are you assuming?
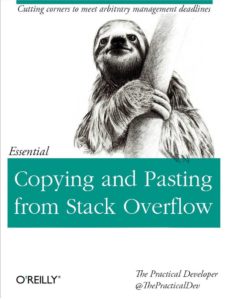
Start by laying out what you know, what you don’t know, and what you’re assuming.
Let’s take a guess at what a binary tree is. Binary means a pair, and a tree is a data structure where objects link to other objects above or below them… So a binary tree is a tree where every object has two child objects?
Say this out loud and ask for clarification: “Is that what you had in mind?” Your interviewer probably wants you to succeed, and is likely to help you, so show your working.
What are the constraints?
What are the success criteria to think about here? Most technical problems can be solved in several ways, and the correct way to solve it depends on the constraints and practical applications of the question.
Start asking about those constraints: “Are we optimizing for anything in particular here? Memory usage or speed?” They may give you a constraint you hadn’t even thought of at this point, and that’s a useful hint.
Test your thinking with examples
Do you have a whiteboard? Great! Start sketching out an example binary tree. What does reversing it even mean? Try a three layer tree and draw out what you’re starting from and where you’re trying to go to. If you’re on the wrong path, you’ll give the interviewer a chance to make a subtle course correction for you at this point.
White-boarding is often a great way of solving technical problems in the real world, and is going to help your brain start to narrow down solutions. We’re trying to show off we know how to solve problems, remember?
What would you need to look up?
You’re not being employed as a walking encyclopedia, so if there are specific things you don’t know or can’t remember, highlight what those are rather than hiding the rest of your knowledge behind small ignorances.
Do you think your method will take an amount of time that’ll grow linearly with the number of elements but don’t remember the technical name for that relationship? That’s fine! Explain it in English.
Does your solution use recursion, and you know there’s a way that some languages optimize recursive operations, but you can’t remember what it’s called? Not a problem! Show your thinking, say what you’re thinking. It’s natural to forget things, but you’re not going to lose many points for having forgotten the term for “tail recursion” if you know it exists.
Say exactly where you’re getting stuck
Does your solution create circular references in a garbage-collected language? Admit it, and be honest that you don’t see the solution, rather than hoping they don’t notice. Is there a feature in that language that would help and you don’t remember what it’s called and how it works? Mention all of that. Your interviewer almost certainly wants you to succeed and will probably give you hints and help you get unstuck, but they’re not psychic. A problem shared is a problem halved.
What are the failure modes
What should you do if there’s a node “missing” in the binary tree? What should you do if you’re given a tree with a single node? Think about what unusual or erroneous input and starting conditions would look like, and reason through what you think reasonable solutions would be. Some interviewers won’t care what behaviour you choose but will be glad you’re thinking about it, and some will want it solved in a certain way.
Thinking about edge-cases is also a trait of an experienced developer, and you’re using this whole process to show that you’re an experienced developer!
Please show your working
Showing your willingness and ability to think is far more important generally than solving a specific problem you’ve been shown.
I will hire the person who had interesting insights in to the problem but got stuck on something because they were nervous, but a person who just sits, assumes, and writes code without discussing the problems they’re trying to solve is a red flag as a future colleague, and that’s assuming they produce a right answer the first time!